Dev C%2b%2b 2d Game Tutorial
- For my class (C Programming), i'm instructed to create a 2D game. Preferably some old school game like pong, snake, breakout, etc. However, i'm not sure what would be a good library for C. I've been looking for tutorials, but all i've found is C. What is a recommended library? I'm using a Macbook Pro (xCode IDE). Any suggestions is appreciated.
- Learn to make a 2D Game in C! Hello developers! Just wanted to provide an update on my video tutorial series, Remaking Cavestory in C. There are now twice as many videos as the first time I posted this, and I wanted to make you all aware of the new content. My main goal for this series is to share my game development knowledge with you.
- Download new video course by Udemy – The complete Game Development with C Learn the basics of C to prepare you for game development programming! What you'll learn Students will be able to start programming in Unreal Engine with C without being intimidated by its advanced techniques. Requirements No programming experience necessary.
- C game coding: Learn to make games using the C programming language. If you have no programming experience but want to get started as fast as possible, or if you need a quick C refresher then this level 1 course is the place to start.
This is the first of a number of tutorials that will guide you through the development of a complete, full-featured game engine. This is part of an ongoing series where we write a complete 2D game engine in C and SFML.
This tutorial shows how to make a C++ pong game in the easiest way possible. The end result are about 150 lines of code in a single source file without any complicated project-, linker- or compiler settings. It uses OpenGL and it works without any crazy math!
Foreword
This pong game will be really easy to make (just as we like it). We will go through the process of setting up our C++ development environment, creating a new project and then writing function by function until our pong game is finished.
Project Setup
Visual Studio 2008
We will use the Visual Studio 2008 Express IDE to make our game. If the link doesn't work anymore, just Google for 'Visual Studio 2008 Express Download'. Newer versions might work too, however older versions would require a lot of complicated setup so 2008 is the way to go.
Once installed, we open Visual Studio and select File->New->Project from the top menu:
Now we select Win32 as Project type and then Win32 Console Application as Template. The last step is to enter a project name and the location where it should be saved:
Dev C 2b 2b 2d Game Tutorial Java
After pressing OK a new window appears where we press Finish. Our project was now created.
Changing _TCHAR* to char**
We see the pong.cpp file right in front of us like this:
Without thinking too much about it, we will change the _TCHAR* thing to char** now:
Note: make sure to also remove the [] brackets behind argv.
If we press F5 (or the green play button), the project should compile fine and a black console window should pop up for a second or so.
OpenGL and GLUT
We want to use the OpenGL graphics library for our project. OpenGL is the way to go when it comes to graphics libraries. It works on all kinds of systems (phones, mac, windows, Linux, ..) and once understood it's a lot of fun to work with.
Dev C 2b 2b 2d Game Tutorial Download
To make our lives easier we will also use the GLUT library. It just provides us with a few more OpenGL functions that would be a bit harder to implement otherwise (things like drawing text or creating a window).
All we have to do to make OpenGL and GLUT work are two things:
1. Download the needed Files, extract them and place them in our project in the same directory that holds the pong.cpp file (in our example it's C:/pong/pong/). Our project directory should then look like this:
2. Include OpenGL and GLUT (and a few more things) at the top of our source code like this:
If we run the project, everything should be fine and the console window should pop up for a second again. Now we can start making the game.
Note: if it says 'freeglut.dll not found' then also copy the freeglut.dll file to the Debug directory.
The Main Method
So far the only function in our project is the main function:
This is the program entry point, which is just the first thing that happens when we run it.
Creating the OpenGL Window
We want to see more than a console popping up for a second, so let's create the OpenGL window. Thanks to the GLUT library, this is incredibly easy:
If we save everything and run the game, we can now see a black window already:
Update and Draw
The main parts of any game loop are the update and draw functions. The update function will calculate the ball and racket movement and things like keyhandling. The draw function will just throw everything at the screen so we can actually see something.
All we have to do is create them and tell GLUT that it should use them. It will then call them automatically all the time.
So let's create the update and draw functions first:
Those functions already contain a few gl and glut function calls. They are just the standard things that we have to do so everything works properly. There is no need to worry about them too much, as they have nothing to do with the gameplay.
So let's tell GLUT to use those functions. We just have to modify our main function again:
We just told GLUT to use our draw function for drawing and our update function for updating. The concept of telling something to call a certain function is called Callback.
So when we run the game now, we still only see a black window. But in the background, update and draw are already called all the time (about 60 times per second).
The 2D Mode and the Color
OpenGL can be used to draw in 2D and in 3D. We want to make a 2D game, so obviously we have to tell OpenGL that. We will create a new enable2D function that will do all the OpenGL configurations that are needed in order to let things appear in 2D. We will just take the function as it is, without worrying too much about whatever crazy math is behind it:
Now let's go back to our main function and call the recently created enable2D function once:
Besides that, we also want to draw our ball and our rackets in white, hence the glColor3f function call in there. The glColor3f function takes three parameters, which are a red, green and blue factor. The factors are between 0 and 1. So if we would want to draw everything in red, we would call glColor3f(1.0f, 0.0f, 0.0f) instead.
That's it for the OpenGL stuff, now it's time to work on the gameplay!
The Score
Let's add a score like '1:3' to our game so the players know who is currently winning.
At first we will declare two score variables just where we declared our window width and height variables. We will have one score for the left player (the one with the left racket) and one for the right player (the one with the right racket):
Now we have a score, but we still have to draw it in order to see something. Again GLUT makes our lives easier here. Drawing a text on the screen works as simple as that:
The code draws the text at the position (x, y). Here is how the coordinates work in OpenGL:
This means that the point (0, 0) (which means that x is 0 and y is 0) is at the bottom left of the window. A point like (500, 0) would be at the bottom right, a point like (0, 500) would be at the top left and a point like (500, 500) would be at the top right of the window.
So let's use our drawText function in our draw function in order to draw our score:
This draws a score like '1:3' at the top center of the window (that's why the x coordinate is roughly width/2 and the y coordinate is roughly height). Since we want to make a game in C++, we have to convert our integer score values to a string in order to use it with our drawText function. We did this with int2str(score). Here is the int2str function, feel free to use it any time you want to convert a integer value to a string:
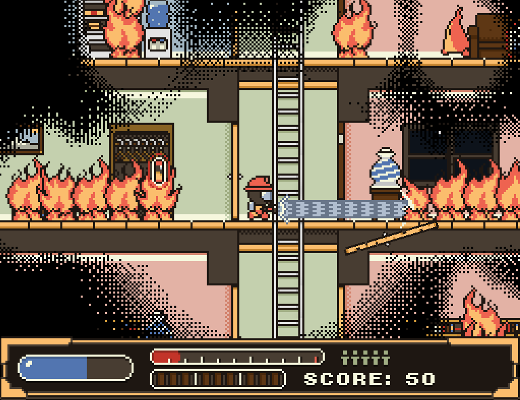
Note: if this throws an error when compiling, make sure you included sstream at the top.
We really want to see something now, so let's run the program. Here is how it looks:
Awesome, seems like all the OpenGL and GLUT stuff was worth it.
The Rackets
Each player needs a racket. One at the left area of the window and one at the right area. Let's implement it!
Racket Variables
We need a few variables again. We have to save the position and the size of each racket. But to save us some work, we will only save the size once instead of saving it for each player:
Note: add this to our variables area again at the top, where we also stored the window size and the score.
As we can see, the left racket should be at (10, 50) which is at the left of the screen and the right racket should be at (width - racket_width - 10, 50) which is at the right of the screen. We used width - racket_width in order to always have it at the right, independent of the window width (If we would change the window width now, the racket would still be perfectly at the right).
Drawing the Rackets
Again, we actually want to see something. The rackets are just simple rectangles. We can draw a rectangle in OpenGL like this:
The function just tells OpenGL to begin drawing a quad, then it tells OpenGL the four points of it via glVertex2f(x, y) and then it tells OpenGL that we are done by calling glEnd().
That's all there is too it. Lines, triangles and all kinds of other shapes can be drawn almost the same way.
So let's go back to our draw function and use the recently created drawRect function in order to draw our two rackets:
Dev C 2b 2b 2d Game Tutorial For Beginners
There really isn't anything complicated to it, just two calls to drawRect with the racket variables. Serial key do windows 8.1 pro 64 bits. If we press run, we can already see where the whole thing is going:
Racket Controls
It's kinda boring to play pong without the ability to move the rackets up and down, so let's add some keyboard handling.
We will use the function GetAsyncKeyState to find out if a key is pressed or not. We will check the W / S keys (for left racket controls) and UpArrow / DownArrow keys (for right racket controls). If they were pressed, we want to increase / decrease the racket's y position.
We declared a racket_speed variable before, this will be the factor by which the racket's y position is increased or decreased. It's really simple, we just add a keyboard function that checks if the keys are down and then call it once every time the game gets updated (in our already existing update function):
Note: something like a += b is just a shorter version of a = a + b.
If we try to run this code we get errors like 'VK_W: undeclared identifier'. For some reason we have to define the values of the W and S key manually, it's really easy though. We just copy them from the Virtual Keycodes site and add this code to the top of our source code (for example where we defined our window size):
If we run it now, we can move the rackets up and down perfectly!
The Ball
Ball Variables
As usual we need a few variables to represent the ball. It needs a position (x and y as usual) which is centered in the screen, a fly direction (dir_x and dir_y), a size and a certain fly speed:
Let's talk about the ball_dir for a second. The ball_dir_x and ball_dir_y variables describe in which direction the ball is currently flying. For example, if x is -1 and y is 0, this means that the ball is flying straight towards the left. If x and y would be 1 this would mean that the ball is flying towards the top-right. Please take a look at the OpenGL Coordinates picture above if there is anything unclear about this.
Drawing the Ball
We already have a drawRect function so we might as well use it to draw the ball. All we have to do is add the following code to our draw function (where we already draw the rackets and the score):
This draws the ball centered at its position with its size. If you find that code to complicated, feel free to use the following less exact but simpler one:
If we press run, we can see the ball already:
Let the Ball fly
Since we already have the ball's fly direction all set up in our ball_dir_x and ball_dir_y variables, we don't have to do much in order to make it fly to the current direction.
To keep everything nice and clear we will add a new function: updateBall which moves the ball a bit into its direction in each update call:
It simply increases the ball's position by its direction multiplied with the speed. No crazy math to it, just one multiplication and one addition.
Reminder: the update function is called about 60 times per second because we set it up as a callback in the GLUT library previously.
Note: as mentioned before, a += b is just a fancier way of doing a = a + b.
Ball Collisions
Alright, the last part of our game takes a slightly bit more math, but still nothing that we didn't learn in school already.
We have to find out if the ball did hit the left or right racket, left or right wall and top or bottom wall. Here is what we do in each case:
- Collision with left racket: let it fly to the right and set the y direction depending on where it hit the racket (so it also flies up and down and not just to the left and to the right).
- Collision with right racket: same as left racket, just to the opposite direction.
- Collision with left wall: increase right player's score and reset ball to the center.
- Collision with right wall: increase left player's score and reset ball to the center.
- Collision with top wall: invert the ball's y fly direction).
- Collision with bottom wall: invert the ball's y fly direction).
Let's just jump into it all at once and get through it. Here is our modified updateBall function:
It's really simple actually. Every time we just check the ball's x and y values to see if it's inside the left racket, inside the right racket, above the top wall, below the bottom wall, left of the left wall or right of the right wall. Then as explained above, we change directions, increase scores and so on.
We also used a t variable at the racket collision parts. This one just specifies where exactly the racket was hit, so we can change the ball's outgoing direction depending on where the racket was hit.
Then there is the vec2_norm function call at the bottom. We need it because we modified the ball_dir_x and ball_dir_y variables before. But in order to have the ball flying at the same speed all the time, the sum of both of those variables should always be exactly 1. That's pretty much what vec2_norm does, it just sets the length of a vector to one.
For example, if we would have something like:
- ball_dir_x = 10.0f
- ball_dir_y = 10.0f
The sum of those two would be 20. Hence the ball would fly pretty fast. After calling vec2_norm, they would be:
- ball_dir_x = 0.5f
- ball_dir_y = 0.5f
Now the sum of those two is exactly 1, while the ratio (or in other words, the fly direction) remains the same.
Here is how our vec2_norm function looks like:
Its not too hard to understand each line of it. It just calculates the squareroot of the sum of the squares of x and y, then divides and multiplies things a bit.
Now don't worry, this is not some crazy magic that we just invented, it's just a formula that can be found in any math book that covers vector math. Make sure to give the whole vector math thing a read one day, because there are a lot of cool things that are used in games every now and then.
If we launch the game, we can now see the ball colliding with the walls and the rackets just as we planned it.
Summary
That's how to make a game in C++. A rather long tutorial, but in the end it's just about 150 lines of code. As usual, our goal was to keep things as easy as possible. There are a lot of improvements that can be done to this game. Examples are sounds, a vector2 class or some nice particle effects and shaders. Maybe even AI for a computer controlled enemy.

Download Source Code & Project Files
The C++ 2D Pong Game source code & project files can be downloaded by Premium members.All Tutorials. All Source Codes & Project Files. One time Payment.
Dev C 2b 2b 2d Game Tutorials
Get Premium today!In this tutorial I will be demonstrating a “quick and easy” way to make a 2D game using OpenGL and a programming language, no engines involved! To follow this tutorial you are expected to have at least a bit of experience using OpenGL and the language you want to use, even if you have only ever gotten as far as making a window and a triangle! I will be using C++ throughout the creation of the game and will be writing this whilst developing the software. I’ll be using Ubuntu 11.04 with the proprietary ATI driver as the platform but it shouldn’t make much difference, the window creation code might be the only difference if the platform being used does not have GLFW.
The whole development process will be split up into 4 stages, each should reach significant milestones leading up to the project’s completion. I’m not going to bother with a design stage, for now the game is going to consist of basic shapes with solid colour. This can be changed later on in development to actual graphics will little effort anyway.
Creating a Window
Hopefully you have GLFW installed somewhere if you are going to use C++ to follow this tutorial. If not, and you are using Ubuntu (maybe Debian, but I don’t know):
Once all of the dependencies are installed, we can create the first two files which will be used in the development process. These files are Main.cpp and Makefile. Main.cpp contains the main() method which will be called when the program is loaded and will initialise the GLFW window, OpenGL and start the main game loop. Makefile is used for making compiling easier on Linux so if you are on windows or using an IDE, you probably won’t want/need one.
Makefile
The contents of the Makefile file are as follows:
SIMPLES! Right? It just calls g++ and compiles Main.cpp into Game and links the two libraries GL and glfw usually found in /usr/lib/gl(fw).o.
Main.cpp
The entry point to the program. It contains the main function which sets up the glfw window and starts the game loop, ready to render objects. The following code does all of the above and is a simple framework on which a game or graphical application can be developed upon.
You should now have two files saved and ready to be compiled! Read through the code if you want, it is all pretty self explanatory. It just creates the window and then goes into an infinite loop, ready to render and update!
To build this on Linux, just type make into a terminal and then run it with ./Game. On windows, do whatever you have to to build it :D.
Once ran, you should be presented with a window like this:
Blank window ready for rendering
It may look a bit bland, but this is the base on which we will create a fully functional game! You can test that OpenGL is actually doing something by changing the code on line 63:
The parameters this function takes are Red value, Green value, Blue value and Alpha value. By changing the first parameter to 1, the window should be red instead of black.